How to Schedule SMS’s and Calls on Twilio
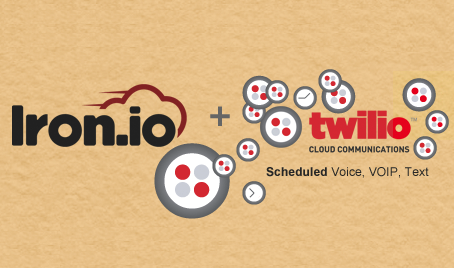
A very common use case for using a service like Twilio is to be able to schedule calls or SMS’s. Maybe you want to schedule a conference call, schedule a text to go out at specific time, or notify all your users about something via SMS every day. Twilio doesn’t have scheduling built in, but here’s an easy way to schedule your Twilio SMS’s and calls using IronWorker. Not only are you able to schedule SMS and calls, IronWorker can allow for some pre-processing to be done, such as sending custom generated messages to users.
Table of Contents
- Install IronWorker
- Create an SMS worker
- Package and upload the worker
- Queue up tasks for the worker
- Queue up tasks to run in the future
- Schedule recurring texts
- Schedule recurring calls
- Conclusion
Note: All of the code in this post is available on Github and I recommend you clone that repository as a starting point rather than copying and pasting from this post. You will need to have an Iron.io account and a Twilio account to run the examples, and this post is meant to quickly take you through the important things without going into setup/configuration, which you can find on this README.
Install IronWorker

Be sure you’ve installed the IronWorker command line (CLI) tool. Documentation for that can be found at, https://dev.iron.io/worker/cli. If you have not installed the dependencies for this script, do so by running:
docker run --rm -v "$PWD":/worker -w /worker iron/ruby:dev bundle install --standalone --clean
Create an SMS worker
Now that the preliminary items are finished, let’s schedule SMS with Twilio through the IronWorker. First, let’s start by creating a simple sms worker. You can write this in any language, but for this post, I’ll be using Ruby. This worker simply sends an SMS via the Twilio API, but you can easily make it do a whole lot more like get data from various places and send a more meaningful message depending on what your application or worker system does. This is sms.rb:
require_relative 'bundle/bundler/setup' require 'twilio-ruby' require 'iron_worker' sid = IronWorker.payload["sid"] token = IronWorker.payload["token"] from = IronWorker.payload["from"] to = IronWorker.payload["to"] body = IronWorker.payload["body"] # set up a client to talk to the Twilio REST API @client = Twilio::REST::Client.new sid, token puts "Sending sms..." r = @client.messages.create( :from => from, :to => to, :body => body ) puts "Twilio response:" p r
You must then fill in your Twilio credentials into your payload file, payload.json:
{ "sid": "YOUR_TWILIO_SID", "token": "YOUR_TWILIO_TOKEN", "from": "TWILIO_PHONE_NUMBER", "to": "NUMBER_TO_SEND_TO", "body": "Hello from IronWorker!" }
Create a Gemfile, or import:
source 'https://rubygems.org' gem 'json' gem 'twilio-ruby' gem 'iron_worker', '>= 3.0.0'
Package and upload the worker
Ok, now that we have our worker files created, we need to package it and upload it. To do that we run this command:
zip -r twilio.zip . iron worker upload --name twilio --zip twilio.zip iron/ruby ruby sms.rb
That will package and upload the sms worker to IronWorker.
Queue up tasks for the worker
Now that we’ve created our sms worker and uploaded it, we can queue up tasks for it. One task or millions of tasks, it doesn’t matter. We simply call our POST task endpoint, so in Ruby, that looks like this:
iron worker queue --payload-file payload.json -delay 60 twilio
Iron.io Serverless Tools
Speak to us to learn how IronWorker and IronMQ are essential products for your application to become cloud elastic.
Queue up tasks to run in the future
Notice the delay option in the code sample above? That is the number of seconds the job will wait before it is executed. If you want to send a text in one hour from now, use the following:
iron worker queue --payload-file payload.json -delay 3600 twilio
Schedule recurring texts
We know how to schedule things to run a single time at some point in the future using the “delay” option, but how do we create a recurring schedule? It’s easy. Just run it as schedule instead of queue:
iron worker schedule -payload-file payload.json -run-every 86400 -run-times 5 twilio
That script will run every 24 hours, with five occurrences (which would be every five days in this case).
Generally, a scheduled task will do more than send out a single SMS. A common use case is to iterate through all your users and queue up a task for each user to send them all a custom SMS. So you might have a “master” worker that queues up a bunch of “slave” tasks.
Schedule recurring calls
All of the above can be switched to calls by just switching from sending texts to making calls using Twilio call API. We will create a file called call.rb:
require_relative 'bundle/bundler/setup' require 'twilio-ruby' require 'iron_worker' sid = IronWorker.payload["sid"] token = IronWorker.payload["token"] from = IronWorker.payload["from"] to = IronWorker.payload["to"] url = IronWorker.payload["url"] # set up a client to talk to the Twilio REST API @client = Twilio::REST::Client.new sid, token puts "Sending sms..." r = @client.calls.create( :from => from, :to => to, :url => url ) puts "Twilio response:" p r
We then need to modify the payload.json file, by replacing the following line:
"body": "Hello from IronWorker!"
With your Twilio Voice API endpoint:
"url": " https://demo.twilio.com/docs/voice.xml"
For more documentation on Twilio’s XML endpoints, visit TwiML For Programmable Voice.
Conclusion

You can now do all kinds of scheduling scenarios for SMS and phone calls with Twilio.
Iron.io can help with your scheduling of worker systems and other business needs. Explore the possibilities with a free 14-day trial of IronWorker, a flexible solution with a wide range of features.
The full code and working examples for this are at: https://github.com/TechDevLab/iron-io-twilio
Unlock the Cloud with Iron.io
Find out how IronWorker and IronMQ can help your application obtain the cloud with fanatical customer support, reliable performance, and competitive pricing.