Build Messaging Between Ruby/Rails Applications with IronMQ
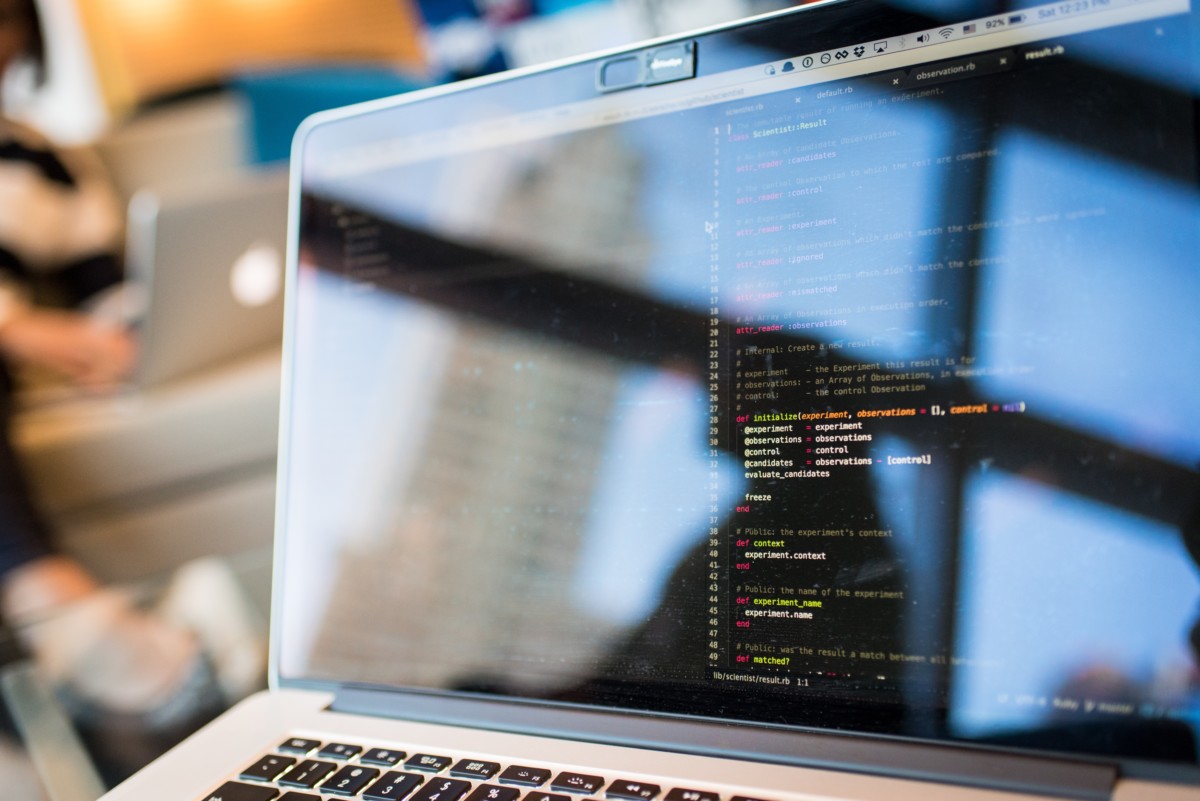
Background job processing is a familiar concept for those working with Ruby/Rails apps, but a far less commonly utilized tool is also far more flexible when it comes to asynchronous execution, and that is a message broker. With a message broker, you can create a message within a given application and then process that message from another application, allowing you to continue executing processes without having to wait for various web applications to respond.
At the architectural level, message brokers provide a number of benefits. First and foremost, they provide fault tolerance and guaranteed delivery. They also enable asynchronous communication thanks to a Publish/Subscribe pattern. Loose coupling and other benefits also come with using a message broker like IronMQ. In this guide, we'll dive deeper into how you can use IronMQ to improve your Ruby/Rails background jobs processing.
Table of Contents
Table of Contents
ToggleInterested In Our Lightning Fast Messaging Queue?
IronMQ is a high-performance messaging queue system with not only fast setup but reliable queues that easily integrate with your application!

When Is a Message Queue Useful?
Developers are all too familiar with laborious tasks and monotonous digital labor when it comes to managing multiple servers or a messaging-specific infrastructure. IronMQ works to make the jobs of these developers a little easier by providing a reliable, lightning-fast means of communicating across services and components.
Ultimately, a message queue will be the best solution if your organization has some kind of processing that happens outside of the flow of your business. A message queue becomes even more necessary if the main flow of your business can go on without waiting for the processing to be done, and especially if the main flow will benefit from being able to go on without waiting for the processing to take place.
Custom event tracking is an extremely common example of this. Simply publish an event, and it will execute without slowing anyone down. Of course, there are countless situations where a message queue will prove beneficial to improving overall efficiency and helping to further optimize business processes.
Common Alternative Solutions for Jobs Processing
To help you determine if a message queue is a right fit for your system, it's important to understand your options.
Using One Database with Multiple Apps
The simplest and most streamlined way to avoid a message queue is simply to point multiple applications to a shared database. Unfortunately, this is a highly flawed alternative. After all, data has validation logic associated with it. This means you either need to duplicate that logic for every application or you have to perform an extraction to get it into your Rails engine gem.
In either case, you'll be dealing with difficult maintenance and still have a very strong coupling across systems. The one use case where this may be a reasonable endeavor is if you have a single read-write application in your systems and then a bunch of read-only apps, but even then this method has its downfalls. Due to these downfalls, it's a readily dismissed option.
Expose the REST API
Rails developers are highly familiar with REST, so exposing the REST API in one Rails application and then calling on it in another may seem like a viable option. In fact, this approach has a number of worthwhile use cases, although it still has many downsides that you must consider. Namely:
- Typically, requests within Ruby are blocking, which means the calling app will need to wait for a response even if it doesn't need it.
- You'll need to ensure that your internal API is not being used externally, which requires authentication.
- This entire process would happen within the server process, so if you're calling an internal API, you're likely using the same server process used for handling "real user" requests, and they should get priority.
- The calling application will have knowledge about the receiving application. Since you need to know which endpoints need to be called on and which parameters should be passed, you've now introduced coupling into your systems.
Ultimately, there may be some situations where exposing the REST API could be useful for your processes, but for most organizations — especially those working at scale — using a message queue is the only option that will provide flexibility, scalability, and reliable speed required to optimize processes.

Popular Message Queue Solutions for Ruby on Rails
Once you've determined that a message queue is the right fit for your organization, the next step will be deciding which message queue service is worth using. There are a number of options on the market, including Sidekiq (as a microservices message processor) and Redis (one of many open source solutions), but some of the most popular are the following.
IronMQ
Running on top of the cloud, IronMQ scales quickly and easily with no need to maintain resources yourself. Outpacing all competitors, IronMQ is one of the fastest solutions on the market. Setup is also extremely simple, with the ability to easily define when and if you want to preserve headers, establish timeouts, default to lazy startups, what to do when a "queue_name" isn't defined, and so on. Best of all, you avoid coupling and dependency.
Apache ActiveMQ
This open-source message queue solution offers an affordable choice but is often overlooked due to its slow speed and performance.
RabbitMQ
With Bunny, the associated Ruby RabbitMQ client, setting up an Advanced Message Queuing Protocol (AMQP) is easy, but it's 10x slower than IronMQ. It's also notorious for having a cluster/HA config process that's simply too complicated to set up and manage.
Choosing the Right Solution
It's important to analyze each one of your options if you're seeking a message queue solution, but without a doubt, IronMQ is one of the fastest options for those prioritizing speed. It's also one of the easiest to scale and one of the most advanced, offering rich features and an excellent developer interface that other options simply can't match.
What Is IronMQ?
IronMQ operates as a message queuing service, designed with the intent of making data management and event flow smoother across applications.
Advantages
Like its competitors, IronMQ supports all major programming languages, including Java, Ruby, Perl, Python, and PHP. It's also the fastest and most powerful message queue option on the market, with reliability and customer support that easily outperforms other solutions.
- Lightning-fast operation.
- Highly scalable across an organization.
- Very developer-friendly.
- Advanced reporting and analytics.
- Easy configuration.
- Delayed delivery options.
- Language agnostic.
- Heroku add-on available.
- JSON compatible.
- Countless Github repositories.
Highly scalable, IronMQ takes the MQ as a Service approach, which helps organizations large and small tackle their background jobs processing with ease.
Iron.io Serverless Tools
Speak to us to learn how IronWorker and IronMQ are essential products for your application to become cloud elastic.
Thanks for subscribing! Please check your email for further instructions.
Quick Messaging Patterns Tutorial
A message broker like IronMQ makes background jobs processing as simple as can be, with only two concepts: Topics and Queues. With modern tools, you'll get to combine these two concepts along with additional features — like IronMQ's pull, push, and long polling support — to get the most out of your systems.
Queue
The base messaging pattern of any setup are queues. Queues enable direct communication between a subscriber and a publisher. The latter (i.e., the "publisher") creates messages while the former (i.e., the "subscriber") reads them, always in the order in which they were received. By queuing up messages, queues automatically organize and keep messages in order of receipt. Once a subscriber reads a message, it leaves the queue, and the next message comes up.
If a queue has multiple subscribers, only one subscriber will get a given message. This is an important feature, as it helps prevent multiple subscribers from trying to execute the same message. Who receives what message depends on your back-end settings and other confirmable options that you can customize to better meet your business's needs.
Topic
With a Topic, one-to-many communication is made possible. In other words, when a queue lines up messages in order and only sends each message to one subscriber, a Topic allows every subscriber to receive every message.
However, a common issue with Topics is that a message can't be recovered for a single listener, like in the instance where the service has disconnected from the associated Topic under which the message was categorized. To help address this common issue, Virtual Topics are often utilized.
Virtual Topic
With a Virtual Topic, the concepts of both Topics and Queues combine to help create a better system. In a Virtual Topic set up, the publisher will send a message to a given Topic, and subscribers will get a copy of the message in their own queue where all the relevant messages are categorized.
Getting Started with IronMQ
Simple projects and infrastructure may be able to get away with using an alternative solution, whether that's one read/write application interfacing with multiple read-only apps or an instance where you choose to expose the REST API for integrations. However, message queues are almost always justified when you need the flexibility and scalability of solving architecture performance issues.
So, if you find yourself in a situation where a message queue would prove beneficial, look no further than IronMQ. As a scalable, lightning-fast solution, IronMQ can help your business take its processes to the next level. Plus, with shared, dedicated, or on-premise performance, IronMQ provides all the flexibility you'd expect from an Iron solution.
Reach Maximum Reliability and Availability with our Tools
Find out how IronWorker and IronMQ can effectively and efficiently help your application with fanatical customer support, reliable performance, and competitive pricing.
